Pandas sum multiple columns of Technology
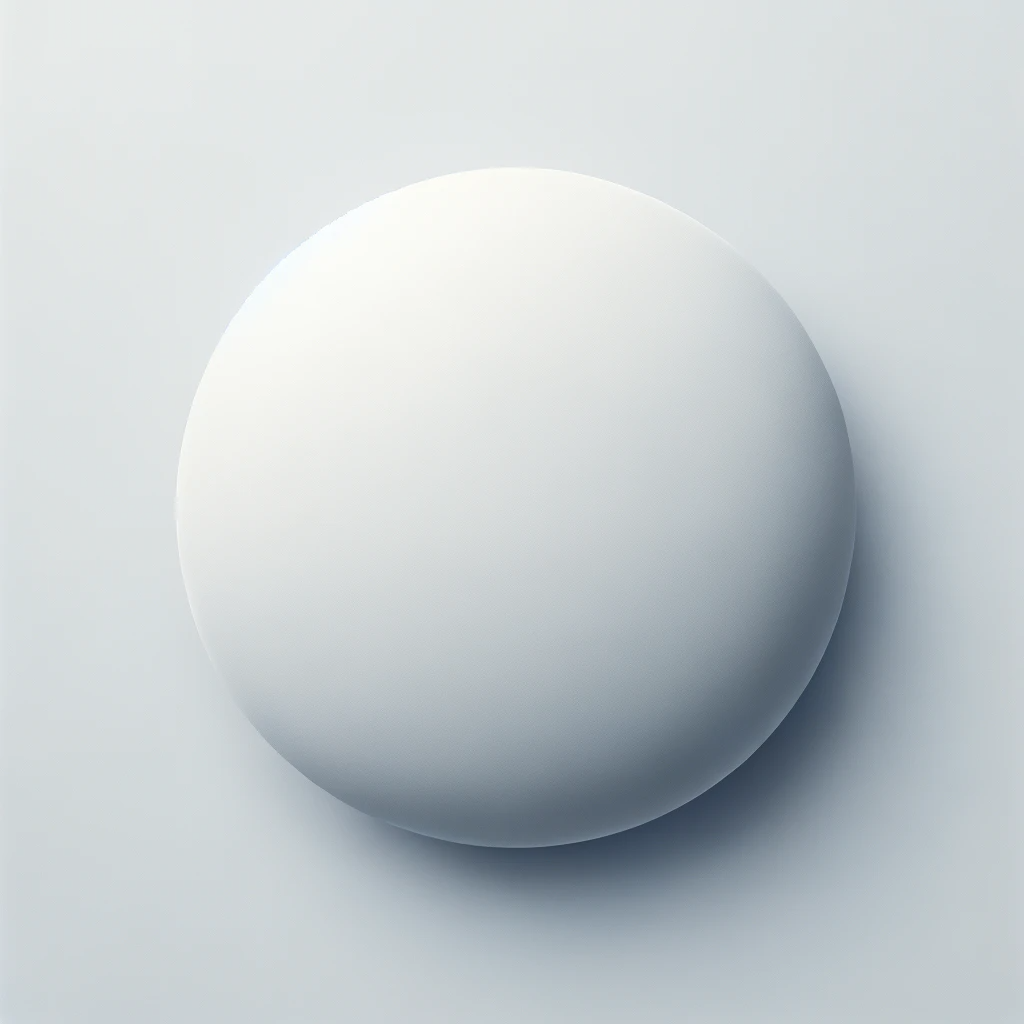
I want to resample daily stock data into monthly stock data. data = yf.download(['AAPL', 'TSLA', 'FB'], '2018-01-01', '2019-01-01')['Close'] for column in data: data ...The reason is dataframe may be having multiple columns and multiple rows. Selective display of columns with limited rows is always the expected view of users. To fulfill the user's expectations and also help in machine deep learning scenarios, filtering of Pandas dataframe with multiple conditions is much necessary.For a single column we’ll simply use the Series Sum () method. # one column. budget['consumer_budg'].sum() 95000. Also the DataFrame has a Sum () method, …Sum columns with nan cell values in Pandas. We can now go ahead and use the fillna () DataFrame method in order to handle cells with missing values and then sum the columns. We will replace the NAN missing values with zeros and sum the columns. This way we can actually skip / ignore the missing values. revenue['total'] = revenue['h1'].fillna(0 ...A paparazzi shot for the ages. The giant panda is vanishingly rare, with fewer than 2,000 specimens left in the wild. But these black-and-white beasts look positively commonplace c...Pandas : Sum multiple columns and get results in multiple columns. 0. ... How to sum multiple columns to get a "groupby" type of output with Python? 1. Pandas groupby.sum for all columns. Hot Network Questions Allow commercial use, but require removal of company nameI have a dataframe which has multiple columns. I'd like to iterate through the columns, counting for each column how many null values there are and produce a new dataframe which displays the sum of isnull values alongside the column header names. If I do: for col in main_df: print(sum(pd.isnull(data[col])))by Zach Bobbitt January 18, 2021. You can use the following syntax to sum the values of a column in a pandas DataFrame based on a condition: df.loc[df['col1'] == some_value, 'col2'].sum() This tutorial provides several examples of how to use this syntax in practice using the following pandas DataFrame: import pandas as pd.Dec 5, 2015 · But transform apparently isn't able to combine multiple columns together because it looks at each column separately (unlike apply). What is the next best alternative in terms of speed / elegance? e.g. I could use apply and then create df['new_col'] by using pd.match, but that would necessitate matching over sometimes multiple groupby columns (col1 and col2) which seems really hacky / would ...Using this function we can get the rolling sum for single or multiple columns of a given Pandas DataFrame. Where n is the size of window. series.rolling(n).sum() function also is used to calculate the rolling sum for Pandas Series. In this article, I will explain how to calculate the rolling sum of pandas DataFrame and series with examples.These solutions are great, but when you have too many columns, you do not want to type all of the column names. So here is what I came up with: column_map = {col: "first" for col in df.columns} column_map["col_name1"] = "sum" column_map["col_name2"] = lambda x: set(x) # it can also be a function or lambda now you can simply doI need to add up the sum of only certain columns: Jan-16, Feb-16, Mar-16, Apr-16 and May-16. I have these columns in a list called months_list. My output should look like the below: A new row called 'Total' should be introduced with a column wise sum for all the columns in my months_list: Jan-16, Feb-16, Mar-16, Apr-16 and May-16. I tried the ...Dec 24, 2018 · import pandas as pd df1 = df.pivot_table(index='Type', columns='Status', values='Number', aggfunc=['sum', 'count'], margins=True, margins_name='Total').fillna(0).drop('Total') # sum count #Status N Y Total N Y Total #Type #A 0.0 400.0 400 0.0 2.0 2 #B 600.0 200.0 800 2.0 1.0 3This can be controlled with the min_count parameter. For example, if you'd like the sum of an empty series to be NaN, pass min_count=1.The sum() method adds all values in each column and returns the sum for each column. By specifying the column axis ( axis='columns' ), the. sum() method searches column-wise and returns the sum of each row.With this method, you find out where column 'a' is equal to 1 and then sum the corresponding rows of column 'b'. You can use loc to handle the indexing of rows and columns: >>> df.loc[df['a'] == 1, 'b'].sum() 15. The Boolean indexing can be extended to other columns. For example if df also contained a column 'c' and we wanted to sum the rows in ...Jan 30, 2015 · With this method, you find out where column 'a' is equal to 1 and then sum the corresponding rows of column 'b'. You can use loc to handle the indexing of rows and columns: >>> df.loc[df['a'] == 1, 'b'].sum() 15. The Boolean indexing can be extended to other columns. For example if df also contained a column 'c' and we wanted to sum the rows in ...Now I want to perform sum of the column operation only on the columns from the list on the dataframe and save that to the dataframe. To put this in prespective, the list of columns ['salary','gross exp'] are money related and it makes sense to perform sum on these columns and not touch any of the other columns ... Find the sum of certain ...Jun 8, 2012 · Define a custom function that will be passed to apply. It implicitly accepts a DataFrame - meaning the data parameter is a DataFrame. Notice how it uses multiple columns, which is not possible with the agg groupby method: def weighted_average(data): d = {} d['d1_wa'] = np.average(data['d1'], weights=data['weights'])We could use GroupBy.sum to get sum by Main ID only where Senior Flag is N, GroupBy.cumsum to get the cumsum Dollar Amt value also when the condition is fulfilled. Series.map in order to assign the value of the sum to the rows where Senior Flag is Y, (assuming that Senior Flag can only take Y or N). df2 = df.sort_values(['Main ID','order'],ascending = [True,False]) m = df['Senior Flag'].eq('N ...Learn how to visualize your data with pandas boxplots. We review how to create boxplots from numerical values and how to customize your boxplot's appearance. Trusted by business bu...Shares of BP have dropped over 6% this year and 25% on the past 12 months, but as oil recovers the oil major could see a tremendous bounce....BP Shares of BP (BP) have dropped over...The output of this code will be `18`, which is the sum of the scores for all rows where the team is 'A'. Example 1: Finding the Sum of One Column Based on the Team. Now let's look at an example that finds the sum of one column based on the team. This is similar to the previous example, but instead of summing a specific set of rows, we ...In pandas, I'd like to create a computed column that's a boolean operation on two other columns. In pandas, it's easy to add together two numerical columns. I'd like to do something similar with logical operator AND. Here's my first try:Tomi Mester. June 18, 2022. Let’s continue with the pandas tutorial series! This is the second episode, where I’ll introduce pandas aggregation methods — such as count(), sum(), min(), max(), etc. — and the pandas groupby() function. These are very commonly used methods in data science projects, so if you are an aspiring data scientist ...Just complementing the solutions presented, if anyone wants to include information from the origin column with the highest value: selected_columns = df.columns. df["C"] = df[selected_columns].apply(max, axis=1) df["from_column"] = df[selected_columns].idxmax(axis=1) The idxmax () method returns a Series with the index of the maximum value for ...pandas.core.groupby.DataFrameGroupBy.sum. #. Compute sum of group values. Include only float, int, boolean columns. Changed in version 2.0.0: numeric_only no longer accepts None. The required number of valid values to perform the operation. If fewer than min_count non-NA values are present the result will be NA.How can I sum the previous row value for amount and another_amount until the last row will have the sum of the entire dataframe? So for example the 2/1 row will have 8 (5+3) for amount and 10 (6+4) for another_amount, then the 3/1 row would have whatever that row contained plus the previous sum of 8 and 10 in their respective columnsBasic Summation. import pandas as pd. import numpy as np. # Creating a simple DataFrame . df = pd.DataFrame({ 'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9] }) # Basic column-wise summation . column_sum = df.sum() print(column_sum) This will output: A 6 . B 15 . C 24 . dtype: int64.I need to add up the sum of only certain columns: Jan-16, Feb-16, Mar-16, Apr-16 and May-16. I have these columns in a list called months_list. My output should look like the below: A new row called 'Total' should be introduced with a column wise sum for all the columns in my months_list: Jan-16, Feb-16, Mar-16, Apr-16 and May-16. I tried the ...Using our previous analogy, it's like sorting the laundry by color and then by fabric type within each color category. Here's how you can group by multiple columns: # Group by both 'Name' and 'City'. multi_grouped = df.groupby(['Name', 'City']) # Display the first entry in each group. for (name, city), group in multi_grouped:2. PySpark Groupby on Multiple Columns. Grouping on Multiple Columns in PySpark can be performed by passing two or more columns to the groupBy() method, this returns a pyspark.sql.GroupedData object which contains agg(), sum(), count(), min(), max(), avg() e.t.c to perform aggregations.. When you execute a groupby operation on multiple columns, data with identical keys (combinations of ...Summing multiple rows having duplicate columns pandas [duplicate] (2 answers) Closed 2 years ago. I'm using Pandas to manipulate a csv file with several rows and columns that looks like the following: ... How do I add third column for the total sum for unique id? like: 'id' 'sum' 1 2.78 2 1.67 3 0.98 4 0.91 5 0.91 7 0.81 I'm pretty sure that it ...2. Add parameter sort=False to groupby for avoid default sorting and aggregate by agg with tuples with new columns names and aggregate functions, last reset_index for MultiIndex to columns: .agg([('count', 'count'),('val', 'sum')]) .reset_index()) name type count val.I have a trouble creating a new column that would sum two existing ones, that I've created form Pivot Tables. ... Sum of columns pandas. Python Help. help. elfzwolf (Greg Florczak) October 9, 2022, 12:29pm 1. Hello. I'm new to python some 6 weeks in, and working on my project. ... It seems like you have multiple column levels, like this:sum_df = df.groupby(by=['year','month'])['score'].sum() But this doesn't look efficient and correct. If I have more than one column need to be aggregate this seems like a very expensive call. for example if I have another column num_attempts and just want to sum by year month as score.0. Assuming you have a pandas dataframe (data), you can subset for specific columns by enclosing the column names in a list. Then you can the use the sum() method to compute the column sums, and then sum again to get the total amount. data[[. '2018 hiv diagnoses', '2018 aids diagnoses',Possibly the fastest solution is to operate in plain Python: Series( map( '_'.join, df.values.tolist() # when non-string columns are present: # df.values.astype(str ...China's newest park could let you see pandas in their natural habitat. Pandas are arguably some of the cutest creatures alive. And you might soon be able to visit China's first nat...I now would like to sort the countries based on the sum of their column and than take the first 2. ... Reordering pandas dataframe based on multiple column and sum of one column. 1. How to sort dataframe by sum of fields grouped by multiple columns. 0. Sort Pandas DataFrame by column. 3.This code will generate a dataframe with hierarchical columns where the top column level signifies the column name from the original dataframe and at the lower level you get each two columns one for the values and one for the counts. def val_cnts_df(df): val_cnts_dict = {} max_length = 0.by Zach Bobbitt January 18, 2021. You can use the following syntax to sum the values of a column in a pandas DataFrame based on a condition: df.loc[df['col1'] == some_value, 'col2'].sum() This tutorial provides several examples of how to use this syntax in practice using the following pandas DataFrame: import pandas as pd.This seems to work, but what if I have multiple columns containing non-integer values? Then I would need to select only certain columns. ... How do I Pandas group-by to get sum? Related. 2. Pivot pandas data and add column. 1. Pivoting multiple columns with pandas. 1. Pandas Pivot Table sum based on other column (as though had two indexes) 1.Sep 12, 2022 · Example 1: Pandas groupby () & sum () by Column Name. In this example, we group data on the Points column and calculate the sum for all numeric columns of DataFrame. Python3. df.groupby(['Points']).sum() Output: Example 2: Pandas groupby () & sum () on Multiple Columns. Here, we can apply a group on multiple columns and calculate a sum over ...This article demonstrates multiple examples to convert the Numpy arrays into Pandas Dataframe and to specify the index column and column headers for the data frame. Example 1: In this example, the Pandas dataframe will be generated and proper names of index column and column headers are mentioned in the function.I have dataframe which has col1-col10, I want to calculate cumulative sum across columns and create new columns on the go i.e. cum_col1-cum_col10. I looked into cumsum(), but that gives final cumulative sum. How to achieve cumulative sum while creating new columns. Dataframe looks like:I think you can use double sum - first DataFrame.sum create Series of sums and second Series.sum get sum of Series: print (df[['a','b']].sum()) a 6 b 12 dtype: int64 print (df[['a','b']].sum().sum()) 18 You can also use: print (df[['a','b']].sum(axis=1)) 0 3 1 6 2 9 dtype: int64 print (df[['a','b']].sum(axis=1).sum()) 18How to add multiple dataframes together. Related. 9. merge 2 dataframes in Pandas: join on some columns, sum up others. 5.Sum multiple multiindex column dataframe. 0. Sum of multi indexed columns pandas. 2. Row sums of dataframe with variable column indexes (Python) 1. Sum specific columns in dataframe with multi index. Hot Network Questions Can "go into" ever be used to mean "used in" or "made of"?Just complementing the solutions presented, if anyone wants to include information from the origin column with the highest value: selected_columns = df.columns. df["C"] = df[selected_columns].apply(max, axis=1) df["from_column"] = df[selected_columns].idxmax(axis=1) The idxmax () method returns a Series with the index of the maximum value for ...I'm looking for the Pandas equivalent of the following SQL: SELECT Key1, SUM(CASE WHEN Key2 = 'one' then data1 else 0 end) FROM df GROUP BY key1 FYI - I've seen conditional sums for pandas aggregate but couldn't transform the answer provided there to work with sums rather than counts. Step 2: Group by multiple columns. First To sum Pandas DataFrame columns (given selectedApply a function to each group independently. Combine the r