Articles on Technology, Health, and Travel
Drop duplicates based on column pandas of Technology
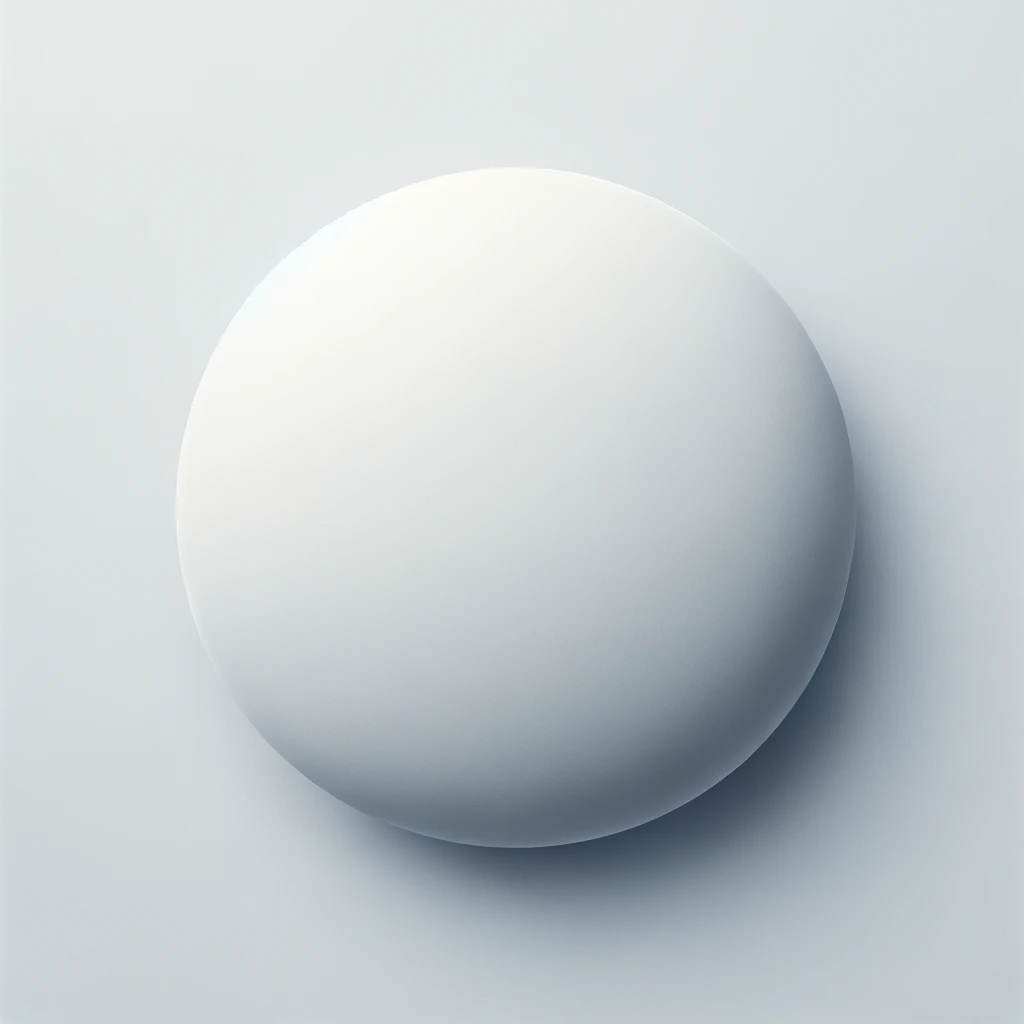
Drop pandas dataframe row based on max value of a column. Ask Question Asked 8 years, 3 months ago. Modified 8 years, 3 months ago. Viewed 29k times 10 I have a Dataframe like so: ... Deleting DataFrame row in Pandas based on column value. 1757. Selecting multiple columns in a Pandas dataframe. 1400.1. Here is a function using difflib. I got the similar function from here. You may also want to check out some of the answers on that page to determine the best similarity metric for your use case. import pandas as pd. import numpy as np. df1 = pd.DataFrame({'Title':['Apartment at Boston','Apt at Boston'],Before dropping duplicates, it's essential to identify them. Pandas provides the duplicated() function for this purpose. This function returns a boolean series, a list-like object where each item corresponds to a row in the DataFrame and indicates whether that row is a duplicate (True) or not (False).This obviously incorrect as it produces an empty dataframe. I've also tried another variation with drop_duplicates that simply deletes all duplicates from 'D', no matter what group it's in. The output I'm looking for is:The idea is to return the index numbers and then you can adress the specific column indices directly. The indices are unique while the column names aren't. def remove_multiples(df,varname): """. makes a copy of the first column of all columns with the same name, deletes all columns with that name and inserts the first column again.How can I drop duplicates based on all columns contained under 'OVERALL' or 'INDIVIDUAL'? So if I choose 'INDIVIDUAL' to drop duplicates from the values of TIP X, TIP Y, and CURVATURE under INDIVIDUAL must all match for it to be a duplicate? And further, as you can see from the table 1 and 2 are duplicates that are simply mirrored about the x ...Here's a one line solution to remove columns based on duplicate column names:. df = df.loc[:,~df.columns.duplicated()].copy() How it works: Suppose the columns of the data frame are ['alpha','beta','alpha']. df.columns.duplicated() returns a boolean array: a True or False for each column. If it is False then the column name is unique up to that point, if it is True then the column name is ...Pandas: drop rows based on duplicated values in a list. 2. dataframe drop_duplicates with subset of columns. 3. ... How to drop duplicates columns from a pandas dataframe, based on columns' values (columns don't have the same name)? Hot Network Questions Merge function in CMar 28, 2021 · What you'll notice is that in this dataframe, there are duplicate "date"s for each "id". This is a reporting error, so what I'd like to do, is go through each "id" and remove one of the duplicate dates rows completely. I would like to KEEP the version of each duplicate date, that had a greater "value". My ideal resulting dataframe would look ...In this article, we will be discussing how to find duplicate rows in a Dataframe based on all or a list of columns. For this, we will use Dataframe.duplicated () method of Pandas.a b. where you're dropping a duplicate row based on the items in 'a' and 'b', without regard to the their specific column. I can hack together a solution using a lambda expression to create a mask and then drop duplicates based on the mask column, but I'm thinking there has to be a simpler way than this: key=lambda x: x[0]) + sorted((x[0], x[1 ...I have 2 equal columns in a pandas data frame. Each of the columns have the same duplicates. A B 1 1 1 1 2 2 3 3 3 3 4 4 4 4 I want to delete the duplicates only from ...Warning: the above solution drop columns based on column name. So a column will be removed even if two columns are not strictly equals, illustration . import pandas as pd import numpy as np data = np.random.randint ... Fast method for removing duplicate columns in pandas.Dataframe;Hi I am droping duplicate from dataframe based on one column i.e "ID", Till now i am droping the duplicate and keeping the first occurence but I want to keep the first(top) two occurrence instead of only one. So I can compare the values of first two rows of another column "similarity_score".I have a dataframe with about a half a million rows. As I could see, there are plenty of duplicate rows, so how can I drop duplicate rows that have the same value in all of the columns (about 80 columns), not just one? df: period_start_time id val1 val2 val3. 06.13.2017 22:00:00 i53 32 2 10.If the values in any of the columns have a mismatch then I would like to take the latest row. On the other question, I did try df.drop_duplicates (subset= ['col_1','col_2']) would perform the duplicate elimination but I am trying to have a check on type column before applying the drop_duplicates methodDropping Duplicates: pandas' DataFrame.drop_duplicates() method allows you to efficiently remove duplicate rows based on identical values in one or more columns.6. You can pass multiple boolean conditions to loc, the first keeps all rows where col 'keep_if_dup' == 'Yes', this is or ed (using |) with the inverted boolean mask of whether col 'url' column is duplicated or not: id url keep_if_dup. to overwrite your df self-assign back:How can I drop duplicates based on all columns contained under 'OVERALL' or 'INDIVIDUAL'? So if I choose 'INDIVIDUAL' to drop duplicates from the values of TIP X, TIP Y, and CURVATURE under INDIVIDUAL must all match for it to be a duplicate? And further, as you can see from the table 1 and 2 are duplicates that are simply mirrored about the x ...Return DataFrame with duplicate rows removed, optionally only considering certain columns. Parameters: subset : column label or sequence of labels, optional. Only consider certain columns for identifying duplicates, by default use all of the columns. keep : {'first', 'last', False}, default 'first'. first : Drop duplicates except ...1. df.drop_duplicates(subset='column_name',keep=False) drop_duplicates will drop duplicated. subset will allow you the specify based on which column you want to determine duplicated. keep will allow you to specify which record to keep or drop.I want to remove duplicates in a column via Pandas. I tried df.drop_duplicates() but no luck. How to achieve this in Pandas?3. Not sure if this is what you means. But if you mean duplicates based on the items, you can collect the items for each customer as a frozenset (if unique), or tuple (if not unique), and then apply drop_duplicates; later on do a filter on the original data frame based on the customer ID. Or if items are not unique and order doesn't matter ...I have a dataframe df1 and column 1 (col1) contains customer id. Col2 is filled with sales and some of the values are missing. My problem is that I want to drop duplicate customer ids in col1 only where the value of sales is missing. I tried writing a function saying: if i[col2] == np.nan: i.drop_duplicates(subset = 'col1') else: return i['col1']: Get the latest Earth-Panda Advanced Magnetic Material stock price and detailed information including news, historical charts and realtime prices. Indices Commodities Currencies...I have a dataframe like this: I would like to drop all rows where the value of column A is duplicate but only if the value of column B is 'true'. The resulting dataframe I have in mind is: I tried using: df.loc[df['B']=='true'].drop_duplicates('A', inplace=True, keep='first') but it doesn't seem to work. Thanks for your help!The keep parameter controls which duplicate values are removed. The value 'first' keeps the first occurrence for each set of duplicated entries. The default value of keep is 'first'. >>> idx.drop_duplicates(keep='first') Index(['lama', 'cow', 'beetle', 'hippo'], dtype='object') Copy to clipboard. The value 'last' keeps the last ...The result after removing the duplicates: Color Shape 0 Green Rectangle 2 Green Square 3 Blue Rectangle 4 Blue Square 5 Red Square 7 Red Rectangle Remove Duplicates under a Specific Column . To remove the duplicates under the Color column using df.drop_duplicates(subset=[“Color”]):The result after removing the duplicates: Color Shape 0 Green Rectangle 2 Green Square 3 Blue Rectangle 4 Blue Square 5 Red Square 7 Red Rectangle Remove Duplicates under a Specific Column . To remove the duplicates under the Color column using df.drop_duplicates(subset=["Color"]):pandas.DataFrame.duplicated. #. Return boolean Series denoting duplicate rows. Considering certain columns is optional. Only consider certain columns for identifying duplicates, by default use all of the columns. Determines which duplicates (if any) to mark. first : Mark duplicates as True except for the first occurrence.4 sample_3 100 20. I want to write something that identifies duplicate entries within the first column and calculates the mean values of the subsequent columns. An ideal output would be something similar to the following: sample_id qual percent. 0 sample_1 30 40. 1 sample_2 15 60.pandas.DataFrame.drop_duplicates. #. Return DataFrame with duplicate rows removed. Considering certain columns is optional. Indexes, including time indexes are ignored. Only consider certain columns for identifying duplicates, by default use all of the columns. Determines which duplicates (if any) to keep. 'first' : Drop duplicates except ...Concatenate two dataframes and drop duplicates in Pandas. Ask Question Asked 4 years, 9 months ago. Modified 4 years, ... Add DataFrame.drop_duplicates for get last rows per type and date after concat. ... Concatenate two dataframes and remove duplicate rows based on column value. 2.Remove duplicate Rows Keep First Occurences. Now, we would like to remove duplicate rows from the DataFrame based on all columns. The first occurrences should be kept. To do this, we use the drop_duplicates() method of Pandas and set the parameter "keep" to "first": df_cleaned = df.drop_duplicates(keep='first') df_cleaned …We can remove or delete a specified column or specified columns by the drop () method. Suppose df is a dataframe. Column to be removed = column0. Code: df = df.drop(column0, axis=1) To remove multiple columns col1, col2, . . . , coln, we have to insert all the columns that needed to be removed in a list.The result from pd.concat([df1, df2], ignore_index=True, sort =False), which clearly have multiple value s in year of 2019 for one type. How should I improve the code? Thank you.I have a data frame df where some rows are duplicates with respect to a subset of columns: . A B C 1 Blue Green 2 Red Green 3 Red Green 4 Blue Orange 5 Blue Orange I would like to remove (or replace with a dummy string) values for duplicate rows with respect to B and C, without deleting the whole row, ideally producing:. A B C 1 Blue Green 2 Red Green 3 NaN NaN 4 Blue Orange 5 Nan NaNChina's newest park could let you see pandas in their natural habitat. Pandas are arguably some of the cutest creatures alive. And you might soon be able to visit China's first nat...I think you need add parameter subset to drop_duplicates for filtering by column id: print pd.concat([df1,df2]).drop_duplicates(subset='id').reset_index(drop=True) id name date 0 1 cab 2017 1 11 den 2012 2 13 ers 1998 3 14 ces 2011 4 4 guk 2007 EDIT: I try your new data and for me it works:So, columns 'C-reactive protein' should be merged with 'CRP', 'Hemoglobin' with 'Hb', 'Transferrin saturation %' with 'Transferrin saturation'. I can easily remove duplicates with .drop_duplicates (), but the trick is remove not only row with the same date, but also to make sure, that the values in the same column are duplicated.Aug 3, 2022 · Pandas drop_duplicates () function removes duplicate rows from the DataFrame. Its syntax is: drop_duplicates(self, subset=None, keep="first", inplace=False) subset: column label or sequence of labels to consider for identifying duplicate rows. By default, all the columns are used to find the duplicate rows. keep: allowed values are …without the third row, as its text is the same as in row one and two, but its timestamp is not within the range of 3 seconds. I tried to define the columns datetime and msg as parameters for the duplicate() method, but it returns an empty dataframe because the timestamps are not identical: mask = df.duplicated(subset=['datetime', 'msg'], keep ...Return DataFrame with duplicate rows removed, optionally only considering certain columns. Parameters: subset : column label or sequence of labels, optional. Only consider certain columns for identifying duplicates, by default use all of the columns. keep : {'first', 'last', False}, default 'first'. first : Drop duplicates except ... I use this rule to filter all rows where column ndropDuplicates keeps the 'first occurreOnce you’ve identified duplicates, removi
Health Tips for Dark souls best strength weapons
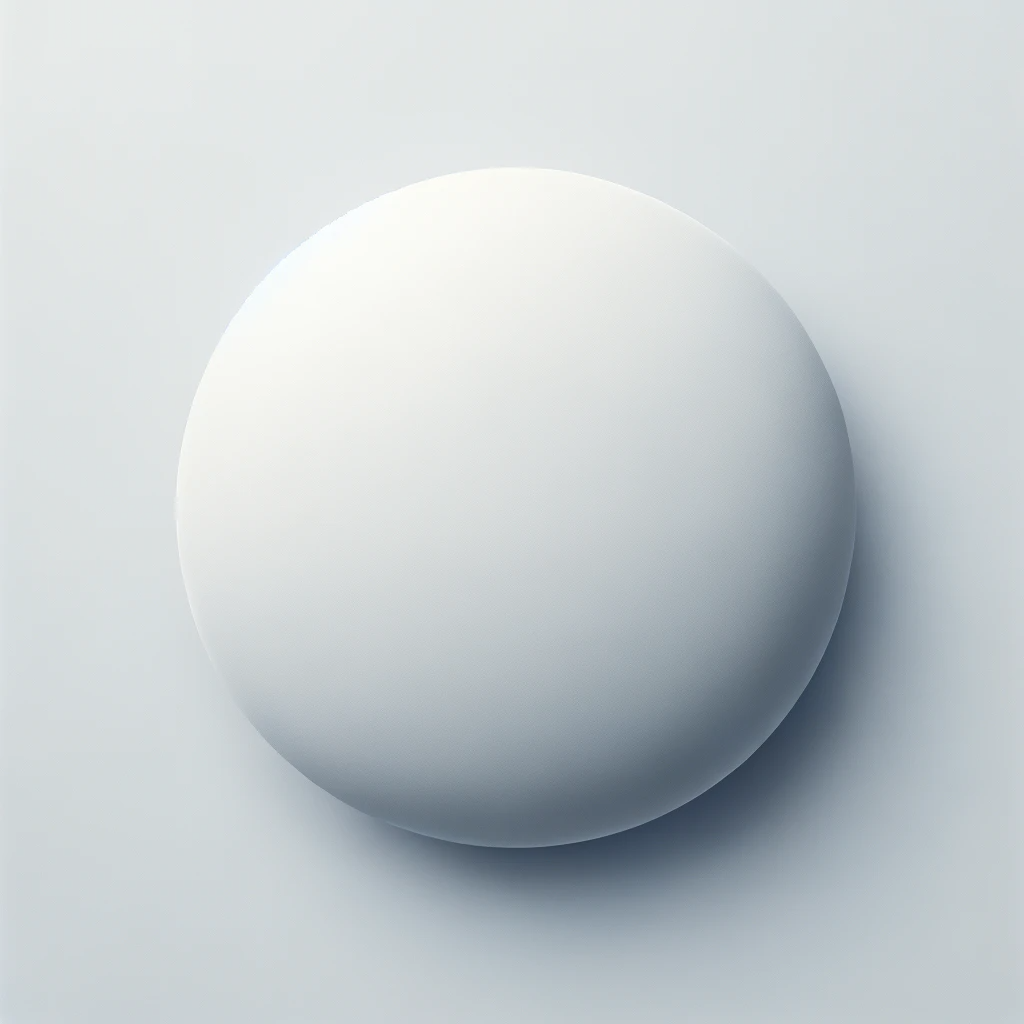
Nov 29, 2017 · Alternative if need remove duplicates rows by B column: df = df.drop_duplicates(subset=['B']) ... Pandas - Removing duplicates based on value in specific column. 2.I can't seem to figure out what would be the best way to go about this? I tried using drop_duplicates but I only know how to use it to select columns for which duplicates will be checked against but I can't figure out how to toggle the keep between first and last depending on the value of "Day" in each row.Aug 20, 2021 · We can use the following code to remove the duplicate ‘points2’ column: #remove duplicate columns df. T. drop_duplicates (). T team points rebounds 0 A 25 11 1 A 12 8 2 A 15 10 3 A 14 6 4 B 19 6 5 B 23 5 6 B 25 9 7 B 29 12 Additional Resources. The following tutorials explain how to perform other common functions in pandas: How to Drop ...You can use pandas duplicated () function to identify the duplicated rows as boolean values series ( True if duplicated) For example, identify duplicated rows based on all columns in the DataFrame, df.duplicated()# output 0False1False2False3Truedtype:bool. The last row of the DataFrame is duplicated as it returns True.You can use the following methods to remove duplicates in a pandas DataFrame but keep the row that contains the max value in a particular column: Method 1: Remove Duplicates in One Column and Keep Row with Max. df.sort_values('var2', ascending=False).drop_duplicates('var1').sort_index() Method 2: Remove Duplicates …1. It's also possible to call duplicated() to flag the duplicates and drop the negation of the flags. df = df[~df.duplicated(subset=['Id'])].copy() This is particularly useful if you want to conditionally drop duplicates, e.g. drop duplicates of a specific value, etc. For example, the following code drops duplicate 'a1' s from column Id (other ...dropDuplicates keeps the 'first occurrence' of a sort operation - only if there is 1 partition. See below for some examples. However this is not practical for most Spark datasets. So I'm also including an example of 'first occurrence' drop duplicates operation using Window function + sort + rank + filter. See bottom of post for example.1. Dataframe df1 drop duplicates row on columns Item and Value. Dataframe df2 keeps the rows where the value between column Group and column Group1 is the same. I want to keep the row where ['Group1'] == df ['Group']. One left thing you need do is to replace values of dataframe df1 with the values of dataframe df2, if their both Item and Value ...You can use the following methods to remove duplicates in a pandas DataFrame but keep the row that contains the max value in a particular column: Method 1: Remove Duplicates in One Column and Keep Row with Max. df.sort_values('var2', ascending=False).drop_duplicates('var1').sort_index() Method 2: Remove Duplicates in Multiple Columns and Keep ...You can use the pandas drop_duplicates () function to drop the duplicate rows based on all columns. You can either keep the first or last occurrence of duplicate …The result from pd.concat([df1, df2], ignore_index=True, sort =False), which clearly have multiple value s in year of 2019 for one type. How should I improve the code? Thank you.Oct 9, 2015 · I want to remove the duplicate rows with respect to column A, and I want to retain the row with value 'PhD' in column B as the original, if I don't find a 'PhD', I want to retain the row with 'Bs' in column B. I am trying to use. df.drop_duplicates('A') with a conditionThis tutorial explains how to drop duplicate rows across multiple columns in pandas, including several examples.Additionally, the size() function creates an unmarked 0 column which you can use to filter for duplicate row. Then, just find length of resultant data frame to output a count of duplicates like other functions: drop_duplicates(), duplicated()==True.How to drop last duplicate row and keep reamining data. ... Make Source and Target column based on consecutive rows. Related. 15. Python Pandas Drop Duplicates keep second to last. 5. Pandas Drop Very First Duplicate only. 2. pandas drop duplicates from a single column while keeping remaining row intact. 4. Pandas: delete consecutive duplicates ...My expected output. col1 col2 col3. I tried below code, but it return me the last row of each group, instead I want to sort by col3 and keep the group with max col3. col1 col2 col3. For Example: Here I want to drop 1st group because 2 < 5, so I want to keep the group with col3 as 5. group = group.reset_index(drop=True)I have a list of items that likely has some export issues. I would like to get a list of the duplicate items so I can manually compare them. When I try to use pandas duplicated method, it only returns the first duplicate. Is there a a way to get all of the duplicates and not just the first one?When using the drop_duplicates() method I reduce duplicates but also merge all NaNs into one entry. ... Python pandas remove duplicate rows that have a column value ...Mar 30, 2020 · I would like to df.drop_duplicates() based off a subset, but also ignore if a column has a specific value.. For example... v1 v2 v3 ID 148 8751704.0 G dog 123 9082007.0 G dog 123 9082007.0 G dog 123 9082007.0 G catI want to remove all duplicates which have a value of 0 on the y column. See my attempt below: ... Pandas drop duplicates where condition. 0. ... Drop duplicates with condition. 1. Drop duplicates based on condition. 3. Filter duplicate rows based on a condition in Pandas. 1. How to get duplicate rows with multiple conditions in ...Here's an example code snippet that demonstrates how to remove duplicate columns from a CSV file using pandas.read_csv(): import pandas as pd # Load CSV file into pandas dataframe df = pd.read_csv('my_data.csv') # Remove duplicate columns df = df.loc[:, ~df.columns.duplicated()] # Display the cleaned DataFrame print(df) Output: Name Age Gender.Jan 26, 2024 · Modified: 2024-01-26 | Tags: Python, pandas. In pandas, the duplicated() method is used to find, extract, and count duplicate rows in a DataFrame, while drop_duplicates() is used to remove these duplicates. This article also briefly explains the groupby() method, which aggregates values based on duplicates. Contents.Pandas drop duplicates across columns. Ask Question Asked 1 year, 10 months ago. Modified 1 year, 10 months ago. Viewed 49 times 2 Good day, I need a way to check ... How to drop duplicate values based in specific columns using pandas? 2. drop duplicates pandas dataframe. 1.3. You can use the function round with a given precision in order to round your df. DataFrame.round (decimals=0, *args, **kwargs) Round a DataFrame to a variable number of decimal places. For example you can apply the round with two decimals by this: df = df.round(2) Also you can apply it on specific columns, for example:The drop_duplicates () function is a built-in function in Pandas that is used to remove duplicate rows from a DataFrame. The function takes several arguments, but the most important ones are: subset: This argument specifies the columns on which the duplicates should be checked. If this argument is not specified, then all columns will be checked ...DBA108642. 2,073 1 20 61. 1. df.drop_duplicates('cust_key') for dropping duplicates based on a single col: cust_key. - anky. Jan 8, 2020 at 16:51. perfect, thank you. I knew it was something small I was missing. If you put this into an answer I'll upvote and accept!image by author. loc can take a boolean Series and filter data based on True and False.The first argument df.duplicated() will find the rows that were identified by duplicated().The second argument : will display all columns.. 4. Determining which duplicates to mark with keep. There is an argument keep in Pandas duplicated() to …How to remove rows with duplicate index values? In the weather DataFrame below, sometimes a scientist goes back and corrects observations -- not by editing the erroneous rows, but by appending aPandas: drop rows based on duplicated values in a list. 2. dataframe drop_duplicates with subset of columns. 3. ... How to drop duplicates columns from a pandas dataframe, based on columns' values (columns don't have the same name)? Hot Network Questions Merge function in CThe easiest way to drop duplicate rows in aI have a data frame df where some rows are duplicates
Top Travel Destinations in 2024
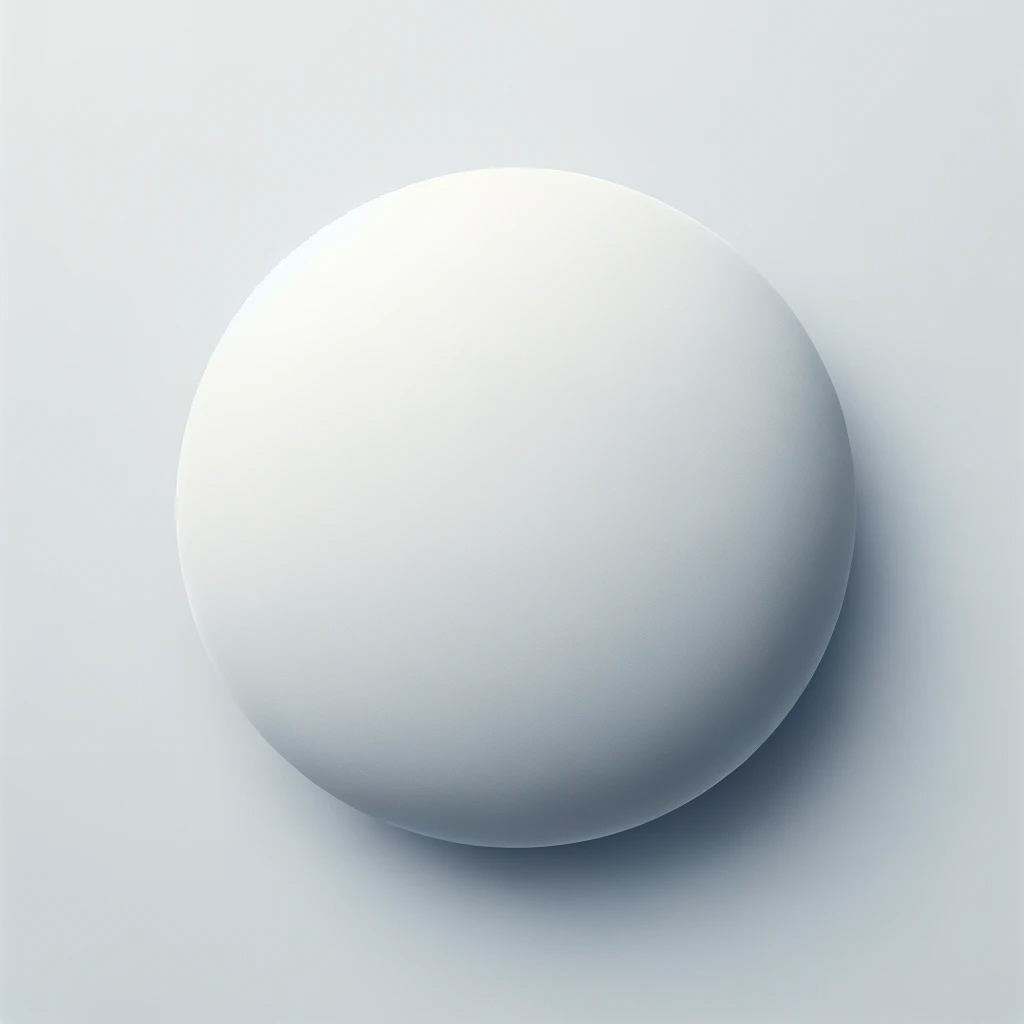
I'd like to drop column B. I tried to use drop_duplicates, but it seems that it only works based on duplicated data not header. Hope anyone know how to do this.To find duplicate columns we need to iterate through all columns of a DataFrame and for each and every column it will search if any other column exists in DataFrame with the same contents already. If yes then that column name will be stored in the duplicate column set. In the end, the function will return the list of column names of the duplicate column. In this way, we can find duplicate ...I'd like to drop rows only if certain values are true. Specifically in my case, I'd like to drop only duplicate rows where Holiday = True and the name and date match. This hopefully gives you an idea of what I'm going for (obviously doesn't work). df = df.drop_duplicates(subset = ['name', 'date', 'holiday' == True], keep='first')Dropping duplicate rows. For demonstration, we will use a subset from the Titanic dataset available on Kaggle. import pandas as pd def load_data(): . df_all = …DBA108642. 2,073 1 20 61. 1. df.drop_duplicates('cust_key') for dropping duplicates based on a single col: cust_key. – anky. Jan 8, 2020 at 16:51. perfect, thank you. I knew it was something small I was missing. If you put this into an answer I'll upvote and accept!3. Not sure if this is what you means. But if you mean duplicates based on the items, you can collect the items for each customer as a frozenset (if unique), or tuple (if not unique), and then apply drop_duplicates; later on do a filter on the original data frame based on the customer ID. Or if items are not unique and order doesn't matter ...Your data contains duplicates, probably because you are only including a subset of the columns. You need something else in your data other than price (e.g. two different days could close at the same price, but you wouldn't aggregate the volume from the two).I would like to remove all duplicates in 'City' column, except if the city name is not in the following list : `["Los Angeles", "New York"]. ... Pandas: Drop duplicates based on row value. 0. How to drop duplicates in column with respect to values in another column in pandas? 1.without the third row, as its text is the same as in row one and two, but its timestamp is not within the range of 3 seconds. I tried to define the columns datetime and msg as parameters for the duplicate() method, but it returns an empty dataframe because the timestamps are not identical: mask = df.duplicated(subset=['datetime', 'msg'], keep ...3. It's already answered here python pandas remove duplicate columns. Idea is that df.columns.duplicated() generates boolean vector where each value says whether it has seen the column before or not. For example, if df has columns ["Col1", "Col2", "Col1"], then it generates [False, False, True]. Let's take inversion of it and call it as column ...pandas.DataFrame.drop_duplicates. #. Return DataFrame with duplicate rows removed. Considering certain columns is optional. Indexes, including time indexes are ignored. Only consider certain columns for identifying duplicates, by default use all of the columns. Determines which duplicates (if any) to keep. 'first' : Drop duplicates except ...To use this method, you simply need to pass in the column names that you want to check for duplicates. For example: df = df.drop_duplicates(subset=['column1','column2']) This will search for any rows that have identical values in the columns 'column1' and 'column2', and will remove them from the dataframe. You can also specify which duplicates ...Is it possible to use the drop_duplicates method in Pandas to remove duplicate rows based on a column id where the values contain a list. Consider column 'three' which consists of two items in a list. Is there a way to drop the duplicate rows rather than doing it iteratively (which is my current workaround).My question is similar to Pandas: remove reverse duplicates from dataframe but I have an additional requirement. I need to maintain row value pairs. For example: I have data where column A corresponds to column C and column B corresponds to column D.. import pandas as pd # Initial data frame data = pd.DataFrame({'A': [0, 10, 11, 21, 22, 35, 5, 50], 'B': [50, 22, 35, 5, 10, 11, 21, 0], 'C': ["a ...2. This indeed is correct answer using in data search and drop. Adding more explanation here. df ['line_race']==0].index -> This will find the row index of all 'line_race' column having value 0. inplace=True -> this will modify original dataframe df.The result from pd.concat([df1, df2], ignore_index=True, sort =False), which clearly have multiple value s in year of 2019 for one type. How should I improve the code? Thank you.In the above example, we create a sample DataFrame with duplicated index using the pd.DataFrame() function. We then drop the duplicated index and reset the index using the reset_index() function. We use the drop_duplicates() function to remove the duplicated index based on the index column and keep the last occurrence of the duplicate rows. Finally, we set the index of the new DataFrame to the ...You can drop duplicate rows on specific multiple columns or all columns using this technique as well. Using functions like DataFrame.drop_duplicates (), DataFrame.apply (), and lambda function with examples, we'll show various techniques to remove duplicate rows from a Pandas DataFrame in this post.The above code does what you want. dfnew=df.append(dfmatch,ignore_index=True) defnew.drop_duplicates(subset=['column1', 'column2', 'column3'], keep = 'first', inplace = True) It adds dfmatch below df creating dfnew. Then it removes the duplicate rows only using column1, 2 and 3 as a subset. It keeps only the first occurrence that corresponds to ...When using the drop_duplicates() method I reduce duplicates but also merge all NaNs into one entry. How can I drop duplicates while preserving rows with an empty entry (like np.nan, None or '' )?The DataFrame.drop_duplicates() function. This function is used to remove the duplicate rows from a DataFrame. DataFrame.drop_duplicates(subset= None, keep= 'first', inplace= False, ignore_index= False) Code language: Python (python) Parameters: subset: By default, if the rows have the same values in all the columns, they are considered duplicates.This parameter is used to specify the columns ...I think you need add parameter subset to drop_duplicates for filtering by column id: print pd.concat([df1,df2]).drop_duplicates(subset='id').reset_index(drop=True) id name date 0 1 cab 2017 1 11 den 2012 2 13 ers 1998 3 14 ces 2011 4 4 guk 2007 EDIT: I try your new data and for me it works:To drop duplicate rows in pandas, you need to use the drop_duplicates method. This will delete all the duplicate rows and keep one rows from each. If you want to permanently change the dataframe then use inplace parameter like this df.drop_duplicates (inplace=True) 3 . Drop duplicate data based on a single column.Apr 12, 2021 · DataFrame with duplicates removed or None if inplace=True. Count unique combinations of columns. Consider dataset containing ramen rating. By default, it removes duplicate rows based on all columns. To remove duplicates on specific column (s), use subset. To remove duplicates and keep last occurrences, use keep.Method 1: str.lower, sort & drop_duplicates. this works with many columns as well. subset = ['firstname', 'lastname'] df[subset] = df ... Drop duplicates based on 2 columns if the value in another column is null - Pandas. 0. Python Pandas drop row duplicates on a column if no duplicate on other column.We can remove or delete a specified column or specified columns by the drop () method. Suppose df is a dataframe. Column to be removed = column0. Code: df = df.drop(column0, axis=1) To remove multiple columns col1, col2, . . . , coln, we have to insert all the columns that needed to be removed in a list.8. The drop_duplicates method of a Pandas DataFrame considers all columns (default) or a subset of columns (optional) in removing duplicate rows, and cannot consider duplicate index. I am looking for a clean one-line solution that considers the index and a subset or all columns in determining duplicate rows. For example, … I'd like to drop column B. I tried to